30 Java Programming Interview Question And Answered for Freshers

Table of Contents
1. Is Java Platform Independent if then how?
Yes, Java is a Platform Independent language. Unlike many programming languages javac compiles the program to form a bytecode or .class file. This file is independent of the software or hardware running but needs a JVM(Java Virtual Machine) file preinstalled in the operating system for further execution of the bytecode.
Although JVM is platform dependent, the bytecode can be created on any System and can be executed in any other system despite hardware or software being used which makes Java platform independent.
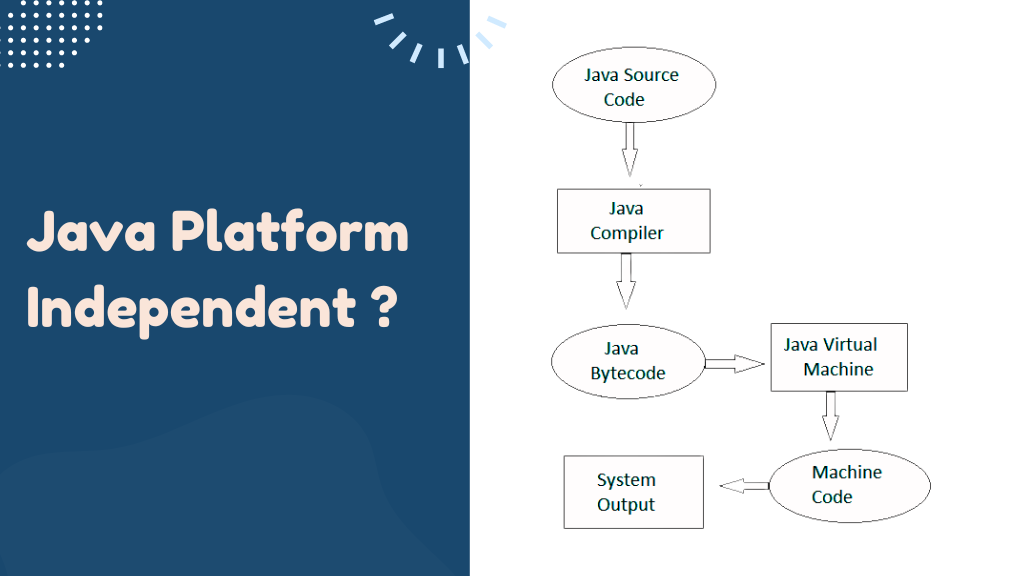
2. What are the top Java Features?
Java is one the most famous and most used language in the real world, there are many features in Java that makes it better than any other language some of them are mentioned below:
Simple: Java is quite simple to understand and the syntax
Platform Independent: Java is platform independent means we can run the same program in any software and hardware and will get the same result.
Interpreted: Java is interpreted as well as a compiler-based language.
Robust: features like Garbage collection, exception handling, etc that make the language robust.
Object-Oriented: Java is an object-oriented language that supports the concepts of class, objects, four pillars of OOPS, etc.
Secured: As we can directly share an application with the user without sharing the actual program makes Java a secure language.
High Performance: faster than other traditional interpreted programming languages.
Dynamic: supports dynamic loading of classes and interfaces.
Distributed: feature of Java makes us able to access files by calling the methods from any machine connected.
Multithreaded: deal with multiple tasks at once by defining multiple threads
Architecture Neutral: it is not dependent on the architecture.

3. What is Java?
Java is an object-oriented, high-level, general-purpose programming language originally designed by James Gosling and further developed by the Oracle Corporation. It is one of the most popular programming languages in the world.
JVM is a program that interprets the intermediate Java byte code and generates the desired output. It is because of bytecode and JVM that programs written in Java are highly portable.
Although JVM is platform dependent, the bytecode can be created on any System and can be executed in any other system despite hardware or software being used which makes Java platform independent.

4. What is the Java Virtual Machine?
JVM is a program that interprets the intermediate Java byte code and generates the desired output. It is because of bytecode and JVM that programs written in Java are highly portable

5. What is a thread in Java?
A thread in Java is a lightweight process that allows concurrent execution of code. Threads can be created by extending the `Thread` class and overriding its `run()` method, or by implementing the `Runnable` interface and passing an instance to a `Thread` object. Threads enable multitasking within a program, improving performance by utilizing CPU resources efficiently.
Here is a short example demonstrating the creation of threads in Java using both the Thread
class and the Runnable
interface:
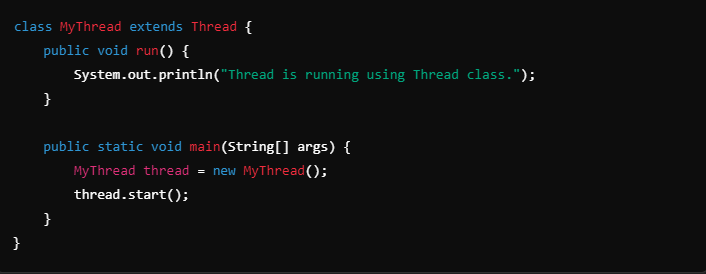
Using the Runnable
Interface:
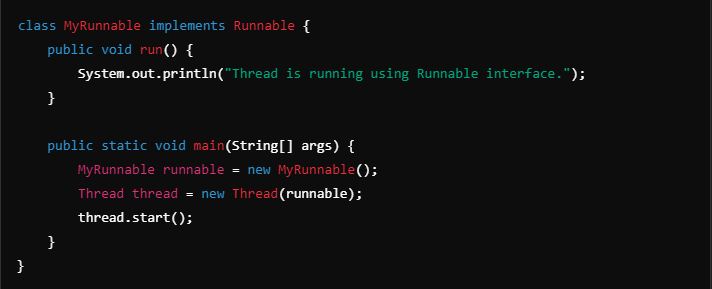
In both examples, the run()
method contains the code that will be executed in the new thread. The start()
method is called to begin the execution of the new thread.
6. What are the differences between WeakHashMap, IdentityHashMap, and EnumMap?
Explanation: This question explores the use of different specialized map implementations in Java. It tests the candidate’s knowledge of memory management (WeakHashMap
), object identity (IdentityHashMap
), and performance optimization with enums (EnumMap
).
7. What is Object-Oriented Programming?
OOPs is a programming paradigm centered around objects rather than functions. It is not a tool or a programming language, it is a paradigm that was designed to overcome the flaws of procedural programming.
Many languages follow OOPs concepts — some popular ones are Java, Python, and Ruby. Some frameworks also follow OOPs concepts, such as Angular.

8. What are static methods and variables?
A class has two sections: one declares variables, and the other declares methods. These are called instance variables and instance methods, respectively. They are termed so because every time a class is instantiated, a new copy of each of them is created.
Variables and methods can be created that are common to all objects and accessed without using a particular object by declaring them static. Static members are also available to be used by other classes and methods.

9. Java is a platform-independent language. Why?
Java does not depend on any particular hardware or software because it is compiled by the compiler and then converted into byte code. Byte code is platform-independent and can run on multiple systems. The only requirement is that Java needs a runtime environment, i.e., JRE, which is a set of tools used for developing Java applications.
10. What are local variables and instance variables?
Variables that are only accessible to the method or code block in which they are declared are known as local variables. Instance variables, on the other hand, are accessible to all methods in a class.
While local variables are declared inside a method or a code block, instance variables are declared inside a class but outside a method. Even when not assigned, instance variables have a value that can be null, 0, 0.0, or false. This isn’t the case with local variables that need to be assigned a value, where failing to assign a value will yield an error. Local variables are automatically created when a method is called and destroyed as soon as the method exits. For creating instance variables, the new keyword must be used

11. What is Overloading?
Overloading is the phenomenon when two or more different methods (method overloading) or operators (operator overloading) have the same representation. For example, the + operator adds two integer values but concatenates two strings. Similarly, an overloaded function called Add can be used for two purposes
- To add two integers
- To concatenate two strings
Unlike method overriding, method overloading requires two overloaded methods to have the same name but different arguments. The overloaded functions may or may not have different return types.
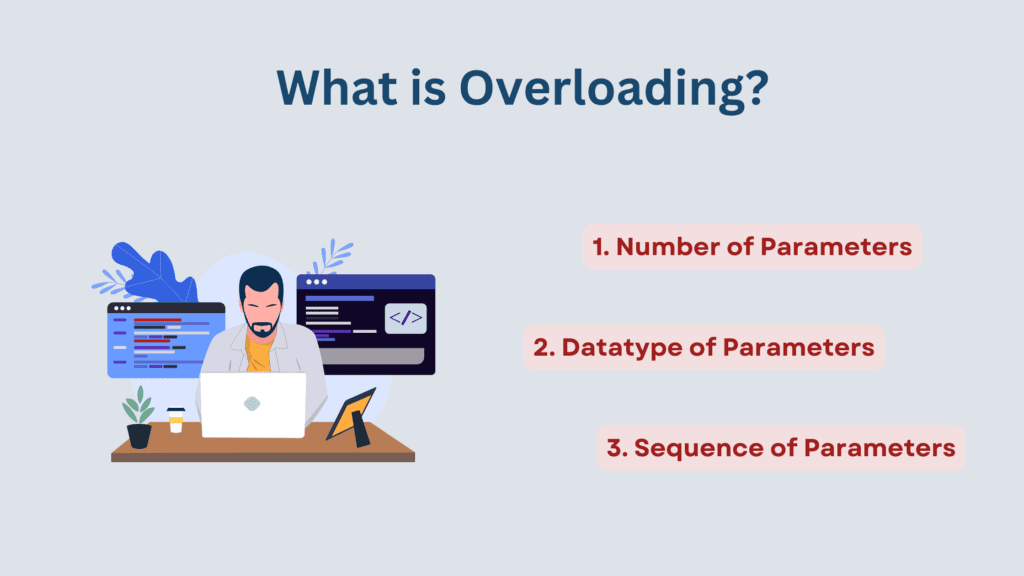
12. What are the differences between String, Stringbuilder, and Stringbuffer?
String variables are stored in a constant string pool. With the change in the string reference, it becomes impossible to delete the old value. For example, if a string has stored a value “Old,” then adding the new value “New” will not delete the old value. It will still be there, however, in a dormant state. In a Stringbuffer, values are stored in a stack. With the change in the string reference, the new value replaces the older value. The Stringbuffer is synchronized (and therefore, thread-safe) and offers slower performance than the StringBuilder, which is also a Stringbuffer but is not synchronized. Hence, performance is faster in Stringbuilder than the Stringbuffer.
13. How is an Abstract class different from an Interface?
There are several differences between an Abstract class and an Interface in Java, summed up as follows:
- Constituents: An abstract class contains instance variables, whereas an interface can contain only constants.
- Constructor and Instantiation: While an interface has neither a constructor nor it can be instantiated, an abstract class can have a default constructor that is called whenever the concrete subclass is instantiated.
- Implementation of Methods – All classes that implement the interface need to provide an implementation for all the methods contained by it. A class that extends the abstract class, however, doesn’t require implementing all the methods contained in it. Only abstract methods need to be implemented in the concrete subclass.
- Type of Methods: Any abstract class has both abstract as well as non-abstract methods. Interface, on the other hand, has only a single abstract method.
14. Please explain Abstract class and Abstract method.
An abstract class in Java is a class that can’t be instantiated. Such a class is typically used for providing a base for subclasses to extend as well as implementing the abstract methods and overriding or using the implemented methods defined in the abstract class.
To create an abstract class, it needs to be followed by the abstract keyword. Any abstract class can have both abstract as well as non-abstract methods. A method in Java that only has the declaration and not implementation is known as an abstract method. Also, an abstract method name is followed by the abstract keyword. Any concrete subclass that extends the abstract class must provide an implementation for abstract methods.

15. What are packages in Java? State some advantages.
Packages are Java’s way of grouping a variety of classes and/or interfaces together. The functionality of the objects decides how they are grouped. Packagers act as “containers” for classes.
Enlisted below are the advantages of Packages:
- Classes of other programs can be reused.
- Two classes with the same can exist in two different packages.
- Packages can hide classes, thus denying access to certain programs and classes meant for internal use only.
- They also separate design from coding.
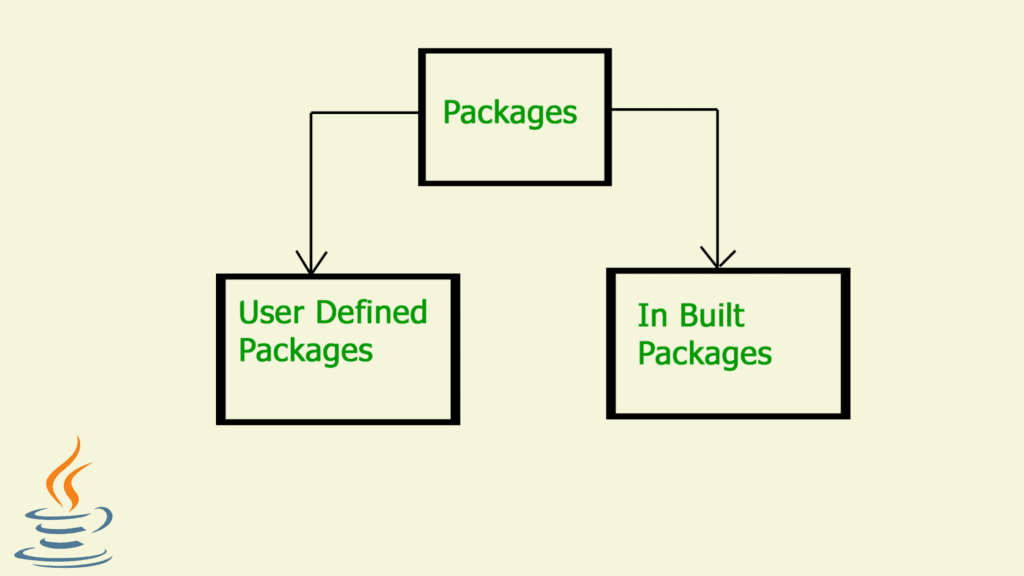
16. Explain the thread lifecycle in Java.
The thread lifecycle has the following states and follows the following order:
- New: In the very first state of the thread lifecycle, the thread instance is created, and the start() method is yet to be invoked. The thread is considered alive now.
- Runnable: After invoking the start() method, but before invoking the run() method, a thread is in the runnable state. A thread can also return to the runnable state from waiting or sleeping.
- Running: The thread enters the running state after the run() method is invoked. This is when the thread begins execution.
- Non-Runnable: Although the thread is alive, it is not able to run. Typically, it returns to the runnable state after some time.
- Terminated: The thread enters the terminated state once the run() method completes its execution. It is not alive now.

17. How will you distinguish processes from threads?
There are several fundamental differences between a process and a thread, stated as follows:
- Definition: A process is an executing instance of a program whereas, a thread is a subset of a process.
- Changes: A change made to the parent process doesn’t affect child processes. However, a change in the main thread can yield changes in the behavior of other threads of the same process.
- Communication – While processes require inter-process communication for communicating with sibling processes, threads can directly communicate with other threads belonging to the same process.
- Control: Processes are controlled by the operating system and can control only child processes. On the contrary, threads are controlled by the programmer and are capable of exercising control over threads of the same process to which they belong.
- Dependence: Processes are independent entities while threads are dependent entities
- Memory: Threads run in shared memory spaces, but processes run in separate memory spaces.
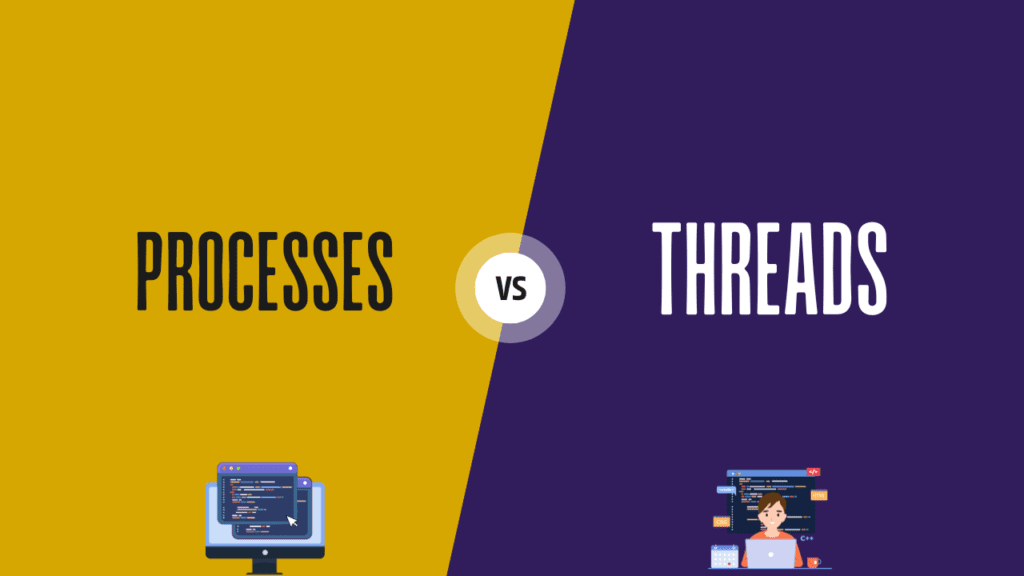
18. How do you make a thread stop in Java?
There are three methods in Java to stop the execution of a thread:
- Blocking: This method is used to put the thread in a blocked state. The execution resumes as soon as the condition of the blocking is met. For instance, the ServerSocket.accept() is a blocking method that listens for incoming socket connections and resumes the blocked thread only when a connection is made.
- Sleeping: This method is used for delaying the execution of the thread for some time. A thread upon which the sleep() method is used is said to enter the sleep state. It enters the runnable state as soon as it wakes up i.e., the sleep state is finished. The time for which the thread needs to enter the sleep state is mentioned inside the braces of the sleep() method. It is a static method.
- Waiting: Although it can be called on any Java object, the wait() method can only be called from a synchronized block.

19. Explain public static void main(String args[ ]) in Java
The execution Java program starts with public static void main(String args[ ]), also called the main() method.
- public: It is an access modifier defining the accessibility of the class or method. Any class can access the main() method defined public in the program.
- static: The keyword indicates the variable, or the method is a class method. The method main() is made static so that it can be accessed without creating the instance of the class. When the method main() is not made static, the compiler throws an error because the main() is called by the JVM before any objects are made, and only static methods can be directly invoked via the class.
- void: It is the return type of the method. Void defines the method that does not return any type of value.
- main: JVM searches this method when starting the execution of any program, with the particular signature only.
- String args[]: The parameter passed to the main method.

20. What is inheritance in Java?
The process by which one class acquires the properties(data members) and functionalities(methods) of another class are called inheritance. The aim of inheritance in java is to provide the reusability of code so that a class has to write only the unique features and the rest of the common properties and functionalities can be extended from another class.
Child Class: The class that extends the features of another class is known as a child class, subclass, or derived class.
Parent Class: The class whose properties and functionalities are used(inherited) by another class is known as the parent class, superclass, or Base class.

21. What is an exception in Java?
An exception is an event, which occurs during the execution of a program, that disrupts the normal flow of the program’s instructions.
When an error occurs within a method, the method creates an object and hands it off to the runtime system. The object, called an exception object, contains information about the error, including its type and the state of the program when the error occurred. Creating an exception object and handing it to the runtime system is called throwing an exception.
After a method throws an exception, the runtime system attempts to find something to handle it. The set of possible “somethings” to handle the exception is the ordered list of methods that had been called to get to the method where the error occurred. The list of methods is known as the call stack.
To create an abstract class, it needs to be followed by the abstract keyword. Any abstract class can have both abstract as well as non-abstract methods. A method in Java that only has the declaration and not implementation is known as an abstract method. Also, an abstract method name is followed by the abstract keyword. Any concrete subclass that extends the abstract class must provide an implementation for abstract methods.

22. What is garbage collection in Java?
Java garbage collection is an automatic process. The programmer does not need to explicitly mark objects to be deleted. The garbage collection implementation lives in the JVM. Each JVM can implement garbage collection however it pleases; the only requirement is that it meets the JVM specification. Although there are many JVMs, Oracle’s HotSpot is by far the most common. It offers a robust and mature set of garbage collection options.
To create an abstract class, it needs to be followed by the abstract keyword. Any abstract class can have both abstract as well as non-abstract methods. A method in Java that only has the declaration and not implementation is known as an abstract method. Also, an abstract method name is followed by the abstract keyword. Any concrete subclass that extends the abstract class must provide an implementation for abstract methods.
23. What is AWT in Java?
The Abstract Window Toolkit (AWT) is Java’s original platform-dependent windowing, graphics, and user-interface widget toolkit, preceding Swing. The AWT is part of the Java Foundation Classes (JFC) — the standard API for providing a graphical user interface (GUI) for a Java program. AWT is also the GUI toolkit for a number of Java ME profiles. For example, Connected Device Configuration profiles require Java runtimes on mobile telephones to support the Abstract Window Toolkit.
24. What is public static void main in Java?
This is the access modifier of the main method. It has to be public so that the java runtime can execute this method. Remember that if you make any method non-public then it’s not allowed to be executed by any program, there are some access restrictions applied. So it means that the main method has to be public. Let’s see what happens if we define the main method as non-public.
When java runtime starts, there is no object of the class present. That’s why the main method has to be static so that JVM can load the class into memory and call the main method. If the main method won’t be static, JVM would not be able to call it because there is no object of the class is present.
Java programming mandates that every method provide the return type. Java’s main method doesn’t return anything, that’s why its return type is void. This has been done to keep things simple because once the main method is finished executing, the java program terminates. So there is no point in returning anything, there is nothing that can be done for the returned object by JVM. If we try to return something from the main method, it will give a compilation error as an unexpected return value.

25. What is Method overloading? Why is it used in Java?
Method overriding is a process in which methods inherited by child classes from parent classes are modified as per requirement by the child class. It’s helpful in hierarchical system design where objects share common properties.
Example: Animal class has properties like fur colour, and sound. Now dog and cat classes inherit these properties and assign values specific to them to the properties.

26. What are the features of Java?
- Java is a pure Object Oriented Programming Language with the following features:
- High Performance
- Platform Independent
- Robust
- Multi-threaded
- Simple
- Secure
27. Tell us something about the JIT compiler.
One of the most important questions asked in the Java interview. JIT(Just-in-time) compiler is a part of Java Virtual Machine and describes a technique used to run a program. It aims to improve the performance of Java programs by compiling byte code into native machine code to run time. It converts code at runtime as demanded during execution. Code that benefits from the compilation is compiled; the rest of the code is interpreted. This improves the runtime performance of programs. For compilation, JVM directly calls the compiled code, instead of interpreting it. The biggest issue with JIT-compiled languages is that the virtual machine takes a couple of seconds to start up, so the initial load time is slower.
There are three types of JIT compilers:
- Pre-JIT: This compiler compiles complete source code into native code in a single compilation cycle and is performed at the time of deployment of the application.
- Econo-JIT: Only those methods are called at runtime are compiled. When not required, this method is removed.
- Normal-JIT: Only those methods called at runtime are compiled. These methods are compiled and then they are stored in the cache and used for execution when the same method is called again.
28. How does the CopyOnWriteArrayList work internally, and when would you use it?
Explanation: CopyOnWriteArrayList
is a thread-safe variant of ArrayList
that is not frequently discussed. This question evaluates the candidate’s understanding of concurrency and the scenarios where CopyOnWriteArrayList
can be beneficial, especially in cases with more reads than writes.
29. Why is Java becoming functional (java 8)?
Java 8 adds functional programming through what are called lambda expressions, which is a simple way of describing a function as some operation on an arbitrary set of supplied variables.
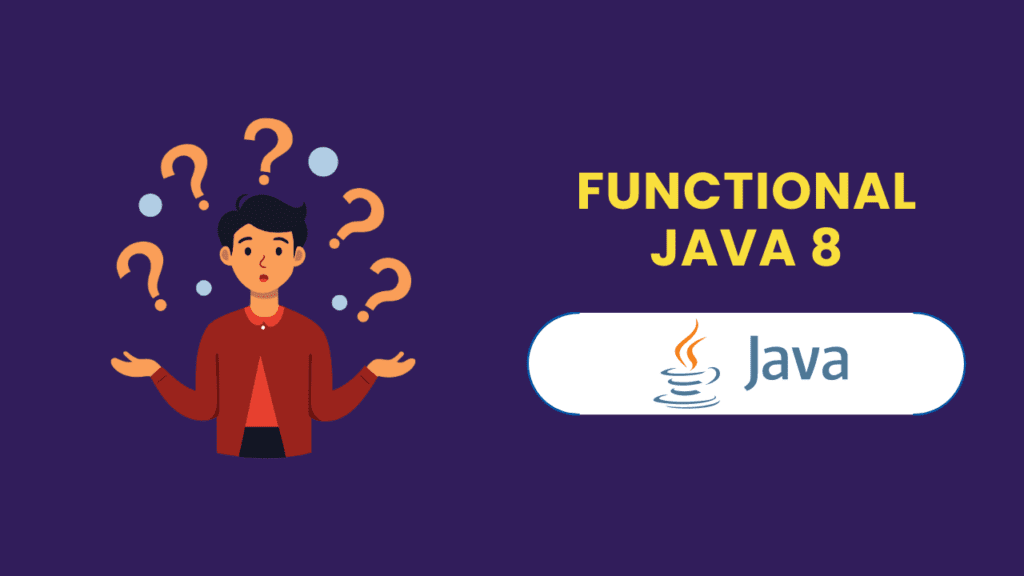
30. How to create a jar file in java?
The basic format of the command for creating a JAR file is:
jar cf jar-file input-file(s)
The options and arguments used in this command are:
- The c option indicates that you want to create a JAR file
- The f option indicates that you want the output to go to a file rather than to stdout
jar-file is the name that you want the resulting JAR file to have. You can use any filename for a JAR file. By convention, JAR filenames are given a .jar extension, though this is not required.
The input-file(s) argument is a space-separated list of one or more files that you want to include in your JAR file. The input-file(s) argument can contain the wildcard * symbol. If any of the “input-files” are directories, the contents of those directories are added to the JAR archive recursively.
The c and f options can appear in either order, but there must not be any space between them.